private static int RandomNumberGenerator(int aStart, int aEnd, Random aRandom){ if ( aStart > aEnd ) { throw new IllegalArgumentException("Start cannot exceed End."); } long range = (long)aEnd - (long)aStart + 1; long fraction = (long)(range * aRandom.nextDouble()); int randomNumber = (int)(fraction + aStart); return randomNumber; }
Friday, November 26, 2010
Random number generator
Pop-ups
function post_to_url(path, params, method) { method = method || "post"; // Set method to post by default, if not specified. //Here we are creating a form tag and adding attributes to it var form = document.createElement("form"); form.setAttribute("method", method); form.setAttribute("action", path); form.setAttribute("target", "
NameofthePopup
"); //Creating input tag and adding attributes to it for(var key in params) { var hiddenField = document.createElement("input"); hiddenField.setAttribute("type", "hidden"); hiddenField.setAttribute("name", key); hiddenField.setAttribute("value", params[key]); //adding input tags to from tag. form.appendChild(hiddenField); } document.body.appendChild(form); // Not entirely sure if this is necessary window.open('URL',"
NameofthePopup
","width=670,height=680,toolbar=no,location=no,directories=no,status=no,menubar=no,resizable=no"); form.submit();// submitting the form }
- In the above function we are using the Post method to send the parameters.
- params should be in follwoing formate(inside the flower brackets):{Arg1:'Arg1Value',Arg2:'Arg2Value'}
Sending an Email
java.util.Properties props = new java.util.Properties();
props.put("mail.smtp.host", "here you have to give the smtp host address");
props.put("mail.smtp.port", "smtp port number, it may or may not required");
Session session = Session.getDefaultInstance(props, null);
// Construct the message
try {
Message msg = new MimeMessage(session);
String emailfrom=request.getParameter("emailfrom");
String emailto=request.getParameter("emailto");
String comment="Hi there";
msg.setContent(comment,"text/html");
msg.setFrom(new InternetAddress(request.getParameter("emailfrom")));
msg.setRecipients(Message.RecipientType.TO,InternetAddress.parse(request.getParameter("emailto")));
msg.setSubject("Enter the subject here");
msg.setSentDate(new Date());
// Sends the message
Transport.send(msg);
response.getWriter().print("success");
} catch (AddressException e) {
// TODO Auto-generated catch block
response.getWriter().print("failed");
e.printStackTrace();
} catch (MessagingException e) {
// TODO Auto-generated catch block
response.getWriter().print("failed");
e.printStackTrace();
}
Sunday, November 21, 2010
SAX Example
Sample s=new Sample();
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
saxParser.parse("Have to give some class object(consider as Sample.java)", inputStream);
inputStream.close();
public class Sample extends DefaultHandler{
public void startElement(String uri, String localName, String qName,Attributes attributes) throws SAXException
{
// This function invokes when parser reads the starting tag
// qName is element name
// below command gets the value of the Attribute "name"
attributes.getValue("name");
}
public void endElement(String uri, String localName, String qName) throws SAXException {
// This function is invoked when the parser reads the ending tag
}
public void characters(char[] chars, int start, int length) throws SAXException {
// Used to read the cdata
String s=new String(chars, start,length); // to read CDATA
}
//Put this line in any of the above method to voluntary exit the
//parsing "throw new DoneParsingException();"
}
How to use Proxy in java
Proxy proxy =null;
URL url;
String decodedXml=null;
DataInputStream inStream=null;
proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress(ProxyAddress, ProxyPort));
// Above line creates proxy with given address and port
url = new URL(getUrl); // you need to give your url here
URLConnection conn = url.openConnection(proxy);// it creates a connection using proxy
String flag;
inStream = new DataInputStream(conn.getInputStream());
Wednesday, November 3, 2010
Char to ascii in javascript
function ascii_value (c)
{
// restrict input to a single character
c = c . charAt (0);
// loop through all possible ASCII values
var i;
for (i = 0; i < 256; ++ i)
{
// convert i into a 2-digit hex string
var h = i . toString (16);
if (h . length == 1)
h = "0" + h;
// insert a % character into the string
h = "%" + h;
// determine the character represented by the escape code
h = unescape (h);
// if the characters match, we've found the ASCII value
if (h == c)
break;
}
return i;
}
References:
Rose, Charlton. "converting between characters and ASCII values." http://sharkysoft.com. 3 Jan 1997. Web. 2 Nov 2010. <http://sharkysoft.com/tutorials/jsa/content/018.html>.
Reading cookies from browser in jsp
<c:forEach items='${cookie}' var='mapEntry'> // reading cookies from any browser
<c:if test='${mapEntry.key eq "AllCookies"}'> // reading from a map
<c:set var="str1" value="${mapEntry.value.value}"/>
<c:forEach var="num" items="${fn:split(str1, ',')}"> // spliting a cookie value
<c:out value='${num}'/>
</c:forEach>
</c:if>
</c:forEach>
Wednesday, October 20, 2010
Monday, October 11, 2010
Buddha's gospels
The poor and the lowly, the rich and the high, are all one, and that all casts unite in this religion as do the rivers in the sea
Never in this world does hatred cease by hatred; hatred ceases by love. And ' let a man overcome anger by kindness, evil by good.'
One may overcome a thousand men in battle, but he who conquers himself is the greatest victor.
Not by birth, but by his conduct alone, does a man become a low-caste or a Brahmin ( i think in his sense educated).
Victory breeds hatred, for the conquered is unhappy.
All that we are is the result of what we have thought.
Once, it is said, he took some dry leaves in his hand and asked his favourite disciple, Ananda, to tell him whether there were any other leaves beside those in his hand. Ananda replied: 'The leaves of autumn are falling on all sides, and there are more of them than can be numbered.' Then said the Buddha: 'In like manner I have fiven you a handful of truths, but besides these there are many thousands of other truths, more than can be numbered.'
Reference:
Jawaharlal, Nehru. THE DISCOVERY OF INDIA. centenary. New Delhi: Oxford University Press, 1989. 1-582. Print.
Never in this world does hatred cease by hatred; hatred ceases by love. And ' let a man overcome anger by kindness, evil by good.'
One may overcome a thousand men in battle, but he who conquers himself is the greatest victor.
Not by birth, but by his conduct alone, does a man become a low-caste or a Brahmin ( i think in his sense educated).
Victory breeds hatred, for the conquered is unhappy.
All that we are is the result of what we have thought.
Once, it is said, he took some dry leaves in his hand and asked his favourite disciple, Ananda, to tell him whether there were any other leaves beside those in his hand. Ananda replied: 'The leaves of autumn are falling on all sides, and there are more of them than can be numbered.' Then said the Buddha: 'In like manner I have fiven you a handful of truths, but besides these there are many thousands of other truths, more than can be numbered.'
Reference:
Jawaharlal, Nehru. THE DISCOVERY OF INDIA. centenary. New Delhi: Oxford University Press, 1989. 1-582. Print.
Friday, October 8, 2010
Calculating Time in milliseconds, in java
long start = System.currentTimeMillis();
float elapsedTimeMillis;
float elapsedTimeMillis;
Put the code here
elapsedTimeMillis = (System.currentTimeMillis()-start);
elapsedTimeMillis =elapsedTimeMillis/1000;
System.out.println("getting xml rowset"+elapsedTimeMillis);
Friday, June 11, 2010
Access properties in jsp
<fmt:message bundle="${link}" key="test"></fmt:message> key is the property we are looking for in the Example.properties file, and ${link} will get its value. Tag library required for this operations is <%@taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
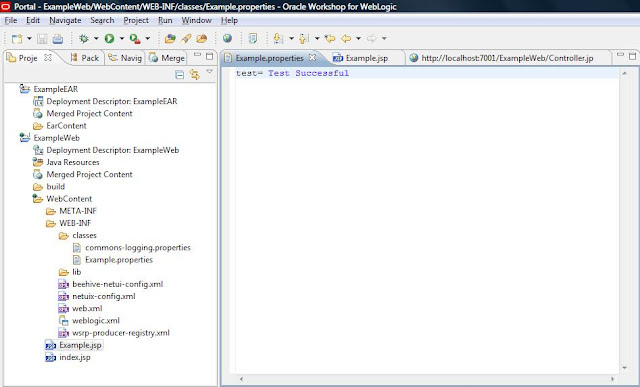
put Example.properties file under WEB-INF/classes/
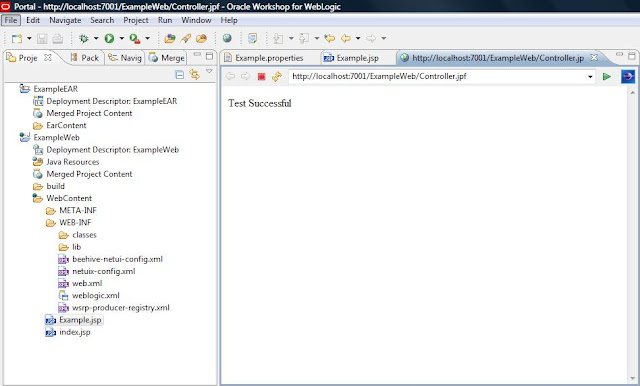
Run Example.jsp you will get the above output
Saturday, April 10, 2010
Page Flows
Controller: Controller are composed of methods and their respective actions. Every incoming request is intercepted by a method in controller and performs business logic and return a Forwards object to an action.
libraries:
import org.apache.beehive.netui.pageflow.PageFlowController;
import org.apache.beehive.netui.pageflow.annotation.Jpf;
Every controller should start with an annotation
@Jpf.Controller
public class controller extends PageFlowController
{}
There are two basic ways of Actions:
1)simple action
2)action method
Simple Actions are class-level annotations. These are declared above class with in controller annotation.
@Jpf.Controller
{
simpleAction={@Jpf.simpleAction(name="" ,path="-----.jsp",[other properties])}
}
public class controller extends PageFlowController
{}
simple action can handle navigation, form submission and form validation. It can not handle decision logic. For decision logic we use action methods.
Action Methods can handle navigation, form submission, form validation, data validation and decision logic. Action Methods return Forward object and decorated with @Jpf.Action annotation.
@Jpf.Action{
forwards={@Jpf.Forward(name="" ,path="-----.jsp",[other properties])}
}
public class controller extends PageFlowController
{}
Monday, March 29, 2010
Use of getServletContext()
When i was doing a project there was a necessity to send an object from one servlet to another servlet. Actually, i was implementing MVC architecture so you can understand in between servlets there is a JSP. So, here is the problem. How to forward an object between servlets?
I came up with an idea of putting the object into session. But, my brother told session is not at all a good idea because it takes lot of server resource. Later, I came up with an idea of using request object and faced trouble sending the objects in request object.
I was thing to implement the logic in different way so that i do not need to send the object to other servlet and i did it with the help of javascript.
Even after the completion of the project, i have question in my mind Is there any other way to send an object between servlets? i found the answer, that is getServletContext()
In servlet1
Resident r=new Resident();
...
...
getServletContext().setAttribute("Obj",r);
In servlet2
Object o= getServletContext().getAttribute("Obj");
Resident r=(Resident)o;
Wednesday, February 3, 2010
tricky question on switch-case
#include
int main()
{
int a=10;
switch(a)
{
case '1': // even if a=1 this case doest not work because it is checking for char '1';
printf("ONE\n");
break;
case '2':
printf("TWO\n");
break;
defa1ut:
printf("NONE\n");
}
return 0;
}
int main()
{
int a=10;
switch(a)
{
case '1': // even if a=1 this case doest not work because it is checking for char '1';
printf("ONE\n");
break;
case '2':
printf("TWO\n");
break;
defa1ut:
printf("NONE\n");
}
return 0;
}
The above program compiles with out any error, but when you run it, it does not give any output
The reason is spelling of key word default is wrong.
Java basic program
Here i am demonstrating a simple program
public class test{public static void main(String args[]){System.out.println("Hello");}}
Save the above file as test.java
Compile the above program: javac test.java
run the program: java test
Output would be: Hello
In Java we write every thing in class. A class should contain a main method which is static and should return void. String args[] is used to take command line arguments.
so far we learned basic program we into some more depth
we will learn different ways of calling a method
public class test{public static void main(String args[]){int i;test1 t=new test1(); // creating a instance of test1i=t.normal(); // calling a method in class test1System.out.println(i);}}class test1 // this class should not be public{public int normal(){try{return 10;}finally{return 20;}}}
Save the above file as test.java
Compile the above program: javac test.java
run the program: java test
Output would be: 20
Above program we wrote two classes, and accessing a method in one class from another class.
public class test{public static int normal(){ // this method should be statictry{return 10;}finally{return 20;}}public static void main(String args[]){int i;test1 t=new test1(); // creating a instance of test1i=t.normal(); // calling a method in class test1System.out.println(i);}}
Save the above file as test.java
Compile the above program: javac test.java
run the program: java test
Output would be: 20
In the above above program we wrote two methods in one class and both method are static. We made normal method static because we want to access it from main method.
Subscribe to:
Posts (Atom)